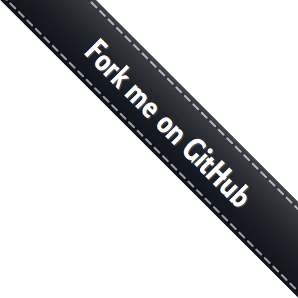
Android documentation
Basics
Doorbell requires a min SDK version of 16.
You can install Doorbell via Maven Central.
Include the following in your dependencies:
implementation 'io.doorbell:android-sdk:0.4.7@aar'
Note The SDK uses AndroidX components, so your app will have to be compatible with it.
In the activity where you want to use Doorbell, you'll need to import the library using:
import io.doorbell.android.Doorbell;
Methods
There are many methods that you can call on the Doorbell object:
setEmail(String email)
addProperty(String key, Object value)
captureScreenshot()
enableNPSRatings()
disableNPSRatings()
resetProperties()
setTags(ArrayList<String> tags)
getDialogBuilder()
show()
And also you can access all the views in the AlertDialog with the following methods:
getMessageField()
getEmailField()
getNPSLabel()
getNPSField()
getNPSScoreLabelLow()
getNPSScoreLabelHigh()
getPoweredByField()
Example
// In your activity
int appId = YOUR-ID-GOES-HERE;
String apiKey = "KEY";
Doorbell doorbellDialog = new Doorbell(this, appId, apiKey); // Create the Doorbell object
doorbellDialog.setEmail("name@example.com"); // Prepopulate the email address field
doorbellDialog.setName("Philip Manavopoulos"); // Set the name of the user (if known)
doorbellDialog.addProperty("loggedIn", true); // Optionally add some properties
doorbellDialog.addProperty("username", 'manavo');
doorbellDialog.addProperty("loginCount", 123);
doorbellDialog.getEmailField().setVisibility(View.GONE); // Hide the email field, since we've filled it in already
doorbellDialog.setTags(new ArrayList<String>(Arrays.asList("tag1", "tag2")));
doorbellDialog.getDialogBuilder().setTitle("Dialog title");
// Capture and send a screenshot of the current activity,
// (only available on certain paid plans)
doorbellDialog.captureScreenshot();
// Enable NPS ratings (only available on certain paid plans)
doorbellDialog.enableNPSRatings();
// Callback for when a message is successfully sent
doorbellDialog.setOnFeedbackSentCallback(new io.doorbell.android.callbacks.OnFeedbackSentCallback() {
@Override
public void handle(String message) {
// Show the message in a different way, or use your own message!
Toast.makeText(context, message, Toast.LENGTH_LONG).show();
}
});
// Callback for when the dialog is shown
doorbellDialog.setOnShowCallback(new io.doorbell.android.callbacks.OnShowCallback() {
@Override
public void handle() {
Toast.makeText(context, "Dialog shown", Toast.LENGTH_LONG).show();
}
});
// Callback for when there is an error, if you want to handle it separately instead of showing a Toast
doorbellDialog.setErrorCallback(new RestErrorCallback() {
@Override
public void error(String errorMessage) {
// Handle message
Toast.makeText(context, errorMessage, Toast.LENGTH_LONG).show();
}
});
doorbellDialog.show();
Material Components Example
// If using Material Components, you can initialize Doorbell like this:
Doorbell doorbellDialog = new Doorbell(this, appId, apiKey, new MaterialAlertDialogBuilder(this));
Note Valid as of SDK version 0.4.0
Screenshots
As shown above in the example, the Android SDK can capture a screenshot of the current activity, and attach it to the message being submitted.
To make the most of this, please ensure that a button to send your a message is included on all activities on which a screenshot would be important.
The ability to capture a screenshot and show Doorbell when the user shakes their device will be released soon.
Shake gesture
You can configure Doorbell to be shown when the device is shaken.
You can configure this in each activity.
Example
import io.doorbell.android.shake.ShakeDetector;
public class MyActivity extends Activity {
int appId = YOUR-ID-GOES-HERE;
String apiKey = "KEY";
protected Doorbell doorbellDialog;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
this.doorbellDialog = new Doorbell(this, appId, apiKey);
this.doorbellDialog.setShakeSensitivity(ShakeDetector.SENSITIVITY_MEDIUM);
}
@Override
protected void onResume() {
super.onResume();
this.doorbellDialog.enableShowOnShake();
}
@Override
protected void onPause() {
super.onPause();
this.doorbellDialog.disableShowOnShake();
}
}